Firma digital de PDF con certificado por software y C++
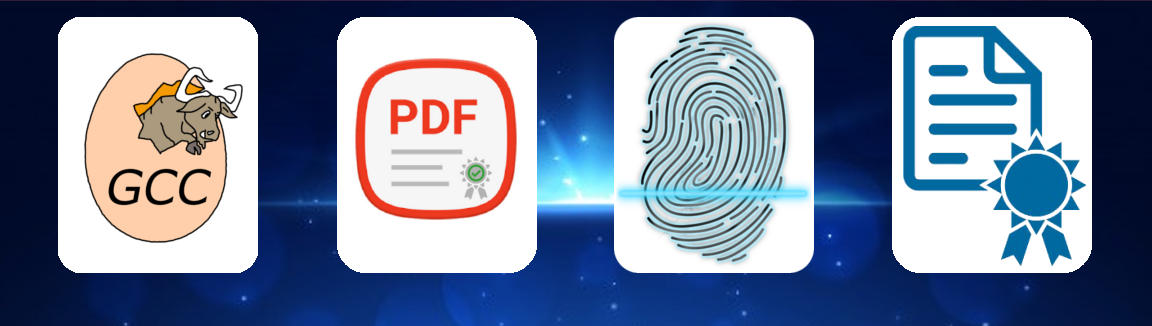
En este tutorial, se verá la implementación de firma digital desde una aplicación escrita en C++, haciendo uso de un par de claves y el certificado disponible en un contenedor PKCS#12.
En el caso de los archivos PDF, se maneja el contenido por secciones, ya que si comparamos el documento original con el firmado, serán diferentes por el contenido de la firma. Sin embargo el contenido propiamente del documento no cambia.
Por lo anterior, el cálculo del HASH se realiza sobre el contenido del PDF como se explica en el siguiente enlace: PDF
El mayor reto al momento de firmar un documento PDF es la manipulación del mismo, por lo que es necesario apoyarse en alguna librería. En nuestro caso se utilizará la librería publicada en: PoDoFo.
Para Debian 10 la librería viene incluída en los repositorios, por lo que solo hará falta ejecutar la siguiente instrucción:
- sudo apt install build-essential libpodofo-dev codeblocks
En caso de utilizar una versión previa a Debian 10, se deberá compilar la versión de libpodofo 0.9.6. Adicionalmente a las dependencias se instaló codeblocks que se utilizará para escribir el programa de manera más amigable, por lo que el primer paso será ingresar a codeblocks y crear un proyecto "Console Application".
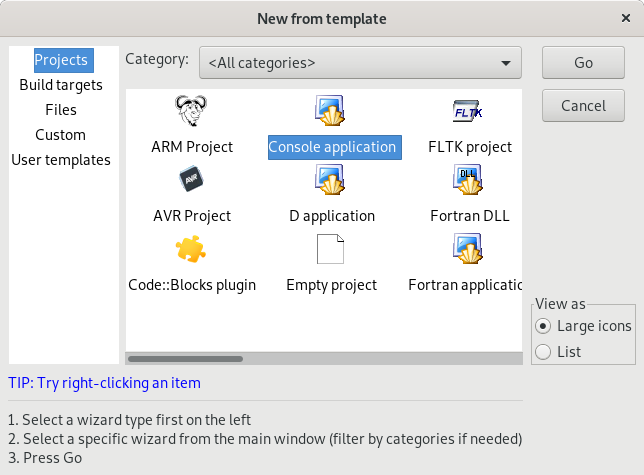
Seguir los siguientes pasos hasta finalizar el asistente.
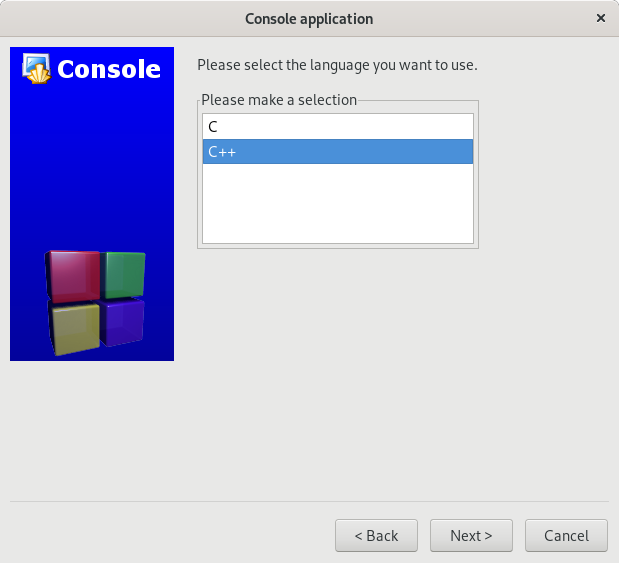
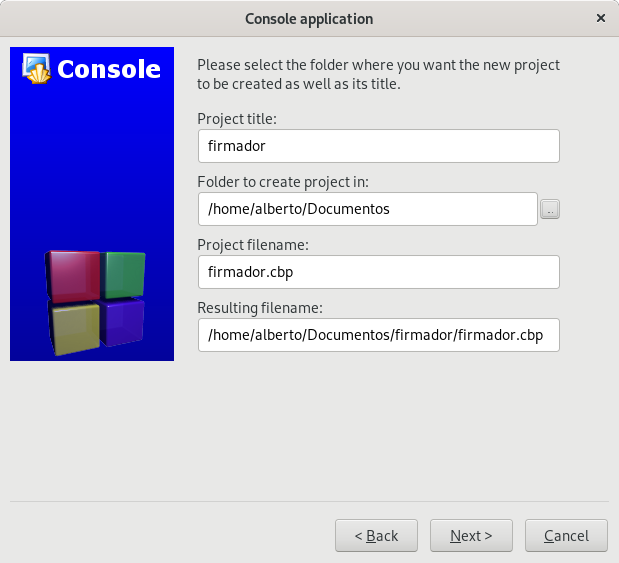
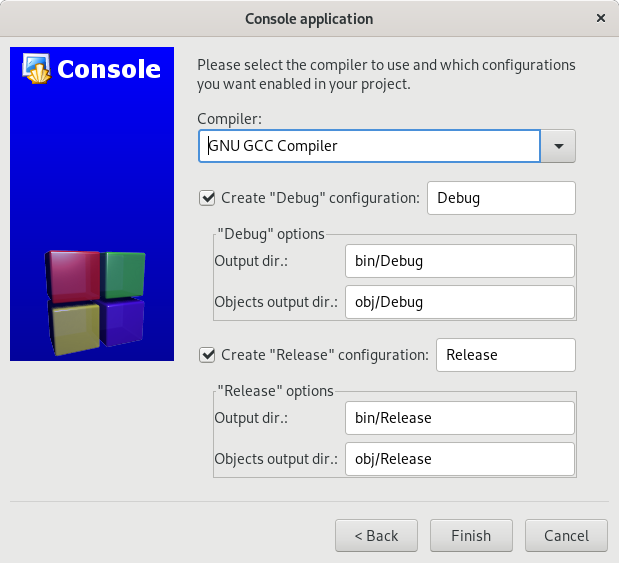
Teniendo el proyecto creado, lo primero será agregar el archivo "podofosign.cpp" que podrá ser reutilizado en otros proyectos para firmar.
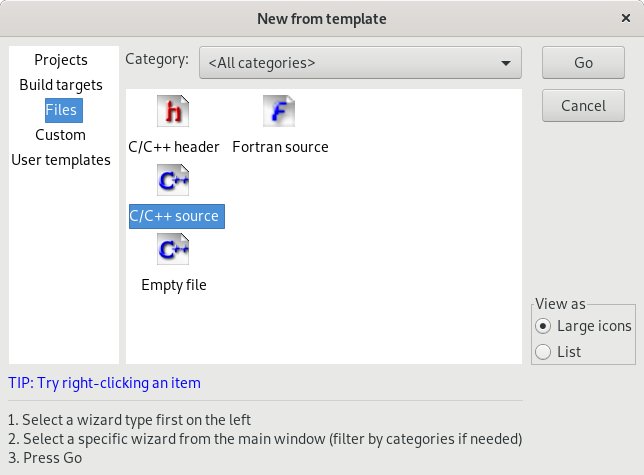
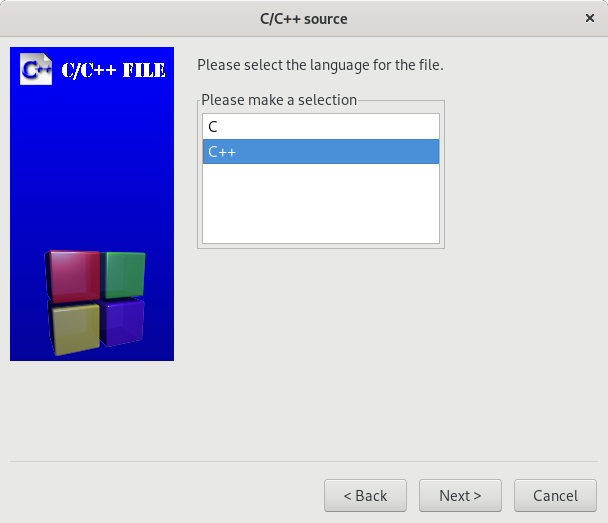
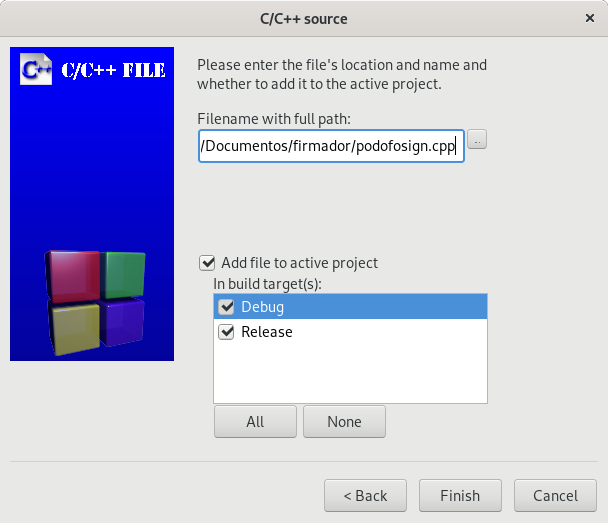
Dentro del archivo agregar las siguientes cabeceras:
- #include <podofo/podofo.h>
- #include <openssl/err.h>
- #include <openssl/pkcs12.h>
- using namespace PoDoFo;
Una vez importadas las cabeceras es necesario crear la siguiente función para calcular el espacio que ocupará la firma dentro del PDF.
- static bool load_cert_and_key(X509** out_cert, EVP_PKEY** out_pkey, pdf_int32& min_signature_size)
- {
- min_signature_size = 0;
- int l;
- if ((l = i2d_X509( *out_cert, NULL )) != -1) {
- min_signature_size += l + 418;
- } else {
- min_signature_size += 3072;
- }
- if ((l = EVP_PKEY_size( *out_pkey )) != -1) {
- min_signature_size += l + 1448;
- } else {
- min_signature_size += 1042;
- }
- return true;
- }
El certificado y llave privada de la función anterior se los obtendrá del archivo pfx mediante la siguiente función.
- void abrir(std::string filePfx, std::string password, X509** cert, EVP_PKEY** pkey)
- {
- FILE *fp = fopen(filePfx.c_str(), "rb");
- if (fp == NULL) {
- throw "No se pudo abrir el archivo.";
- }
- PKCS12 *p12_cert = NULL;
- STACK_OF(X509) *additional_certs = NULL;
- OpenSSL_add_all_algorithms();
- if (d2i_PKCS12_fp(fp, &p12_cert) < 0) {
- fclose(fp);
- throw "Error al leer el archivo.";
- }
- fclose(fp);
- if (PKCS12_parse(p12_cert, password.c_str(), pkey, cert, &additional_certs) == 0) {
- int err = ERR_get_error();
- switch (err) {
- case 218570875:
- case 218542222:
- case 218529960:
- throw "Formato de archvio incorrecto.";
- case 587686001:
- throw "Contraseña incorrecta.";
- default:
- throw "Error desconocido.";
- }
- }
- }
Para verificar que no existan otros campos de firma.
- static PdfObject* find_existing_signature_field(PdfAcroForm* pAcroForm, const PdfString& name)
- {
- if( !pAcroForm )
- PODOFO_RAISE_ERROR( ePdfError_InvalidHandle );
- PdfObject* pFields = pAcroForm->GetObject()->GetDictionary().GetKey( PdfName( "Fields" ) );
- if( pFields )
- {
- if( pFields->GetDataType() == ePdfDataType_Reference )
- pFields = pAcroForm->GetDocument()->GetObjects()->GetObject( pFields->GetReference() );
- if( pFields && pFields->GetDataType() == ePdfDataType_Array )
- {
- PdfArray &rArray = pFields->GetArray();
- PdfArray::iterator it, end = rArray.end();
- for( it = rArray.begin(); it != end; it++ )
- {
- // require references in the Fields array
- if( it->GetDataType() == ePdfDataType_Reference )
- {
- PdfObject *item = pAcroForm->GetDocument()->GetObjects()->GetObject( it->GetReference() );
- if( item && item->GetDictionary().HasKey( PdfName( "T" ) ) &&
- item->GetDictionary().GetKey( PdfName( "T" ) )->GetString() == name )
- {
- // found a field with the same name
- const PdfObject *pFT = item->GetDictionary().GetKey( PdfName( "FT" ) );
- if( !pFT && item->GetDictionary().HasKey( PdfName( "Parent" ) ) )
- {
- const PdfObject *pTemp = item->GetIndirectKey( PdfName( "Parent" ) );
- if( !pTemp )
- {
- PODOFO_RAISE_ERROR( ePdfError_InvalidDataType );
- }
- pFT = pTemp->GetDictionary().GetKey( PdfName( "FT" ) );
- }
- if( !pFT )
- {
- PODOFO_RAISE_ERROR( ePdfError_NoObject );
- }
- const PdfName fieldType = pFT->GetName();
- if( fieldType != PdfName( "Sig" ) )
- {
- std::string err = "Existing field '";
- err += name.GetString();
- err += "' isn't of a signature type, but '";
- err += fieldType.GetName().c_str();
- err += "' instead";
- PODOFO_RAISE_ERROR_INFO( ePdfError_InvalidName, err.c_str() );
- }
- return item;
- }
- }
- }
- }
- }
- return NULL;
- }
Y para crear la firma se utilizará la siguiente función.
- static void sign_with_signer( PdfSignOutputDevice &signer, X509 *cert, EVP_PKEY *pkey )
- {
- if( !cert )
- PODOFO_RAISE_ERROR_INFO( ePdfError_InvalidHandle, "cert == NULL" );
- if( !pkey )
- PODOFO_RAISE_ERROR_INFO( ePdfError_InvalidHandle, "pkey == NULL" );
- unsigned int uBufferLen = 65535, len;
- char *pBuffer;
- while( pBuffer = reinterpret_cast<char *>( podofo_malloc( sizeof( char ) * uBufferLen) ), !pBuffer )
- {
- uBufferLen = uBufferLen / 2;
- if( !uBufferLen )
- break;
- }
- if( !pBuffer )
- PODOFO_RAISE_ERROR (ePdfError_OutOfMemory);
- int rc;
- BIO *mem = BIO_new( BIO_s_mem() );
- if( !mem )
- {
- podofo_free( pBuffer );
- throw "Failed to create input BIO";
- }
- unsigned int flags = PKCS7_DETACHED | PKCS7_BINARY;
- PKCS7 *pkcs7 = PKCS7_sign( cert, pkey, NULL, mem, flags );
- if( !pkcs7 )
- {
- BIO_free( mem );
- podofo_free( pBuffer );
- throw "PKCS7_sign failed";
- }
- while( len = signer.ReadForSignature( pBuffer, uBufferLen ), len > 0 )
- {
- rc = BIO_write( mem, pBuffer, len );
- if( static_cast<unsigned int>( rc ) != len )
- {
- PKCS7_free( pkcs7 );
- BIO_free( mem );
- podofo_free( pBuffer );
- throw "BIO_write failed";
- }
- }
- podofo_free( pBuffer );
- if( PKCS7_final( pkcs7, mem, flags ) <= 0 )
- {
- PKCS7_free( pkcs7 );
- BIO_free( mem );
- throw "PKCS7_final failed";
- }
- bool success = false;
- BIO *out = BIO_new( BIO_s_mem() );
- if( !out )
- {
- PKCS7_free( pkcs7 );
- BIO_free( mem );
- throw "Failed to create output BIO";
- }
- char *outBuff = NULL;
- long outLen;
- i2d_PKCS7_bio( out, pkcs7 );
- outLen = BIO_get_mem_data( out, &outBuff );
- if( outLen > 0 && outBuff )
- {
- if( static_cast<size_t>( outLen ) > signer.GetSignatureSize() )
- {
- PKCS7_free( pkcs7 );
- BIO_free( out );
- BIO_free( mem );
- std::ostringstream oss;
- oss << "Requires at least " << outLen << " bytes for the signature, but reserved is only " << signer.GetSignatureSize() << " bytes";
- PODOFO_RAISE_ERROR_INFO( ePdfError_ValueOutOfRange, oss.str().c_str() );
- }
- PdfData signature( outBuff, outLen );
- signer.SetSignature( signature );
- success = true;
- }
- PKCS7_free( pkcs7 );
- BIO_free( out );
- BIO_free( mem );
- if( !success )
- throw "Failed to get data from the output BIO";
- }
Finalmente la función que hace toda la magia.
- const char* sign(const char *inputfile, const char *filePfx, const char *password)
- {
- EVP_PKEY *pkey;
- X509 *cert;
- abrir(filePfx, password, &cert, &pkey);
- const char *outputfile = "/tmp/signed.pdf";
- const char *reason = "I agree";
- const char *sigsizestr = NULL;
- const char *annot_position = NULL;
- const char *field_name = NULL;
- int annot_page = 0;
- double annot_left = 0.0, annot_top = 0.0, annot_width = 0.0, annot_height = 0.0;
- bool annot_print = false;
- bool field_use_existing = false;
- PdfError::EnableDebug( false );
- int sigsize = -1;
- if( sigsizestr )
- {
- sigsize = atoi( sigsizestr );
- if( sigsize <= 0 )
- {
- throw (std::string("Invalid value for signature size specified (") + sigsizestr + "), use a positive integer, please").c_str();
- }
- }
- if( outputfile && strcmp( outputfile, inputfile ) == 0)
- {
- // even I told you not to do it, you still specify the same output file
- // as the input file. Just ignore that.
- outputfile = NULL;
- }
- #ifdef PODOFO_HAVE_OPENSSL_1_1
- OPENSSL_init_crypto(0, NULL);
- #else
- OpenSSL_add_all_algorithms();
- ERR_load_crypto_strings();
- ERR_load_PEM_strings();
- ERR_load_ASN1_strings();
- ERR_load_EVP_strings();
- #endif
- pdf_int32 min_signature_size = 0;
- if( !load_cert_and_key( &cert, &pkey, min_signature_size ) )
- {
- throw "Error al cargar el certificado y clave.";
- }
- if( sigsize > 0 )
- min_signature_size = sigsize;
- PdfSignatureField *pSignField = NULL;
- PdfAnnotation *pTemporaryAnnot = NULL; // for existing signature fields
- try
- {
- PdfMemDocument document;
- document.Load( inputfile, true );
- if( !document.GetPageCount() )
- PODOFO_RAISE_ERROR_INFO( ePdfError_PageNotFound, "The document has no page. Only documents with at least one page can be signed" );
- PdfAcroForm* pAcroForm = document.GetAcroForm();
- if( !pAcroForm )
- PODOFO_RAISE_ERROR_INFO( ePdfError_InvalidHandle, "acroForm == NULL" );
- if( !pAcroForm->GetObject()->GetDictionary().HasKey( PdfName( "SigFlags" ) ) ||
- !pAcroForm->GetObject()->GetDictionary().GetKey( PdfName( "SigFlags" ) )->IsNumber() ||
- pAcroForm->GetObject()->GetDictionary().GetKeyAsLong( PdfName( "SigFlags" ) ) != 3 )
- {
- if( pAcroForm->GetObject()->GetDictionary().HasKey( PdfName( "SigFlags" ) ) )
- pAcroForm->GetObject()->GetDictionary().RemoveKey( PdfName( "SigFlags" ) );
- pdf_int64 val = 3;
- pAcroForm->GetObject()->GetDictionary().AddKey( PdfName( "SigFlags" ), PdfObject( val ) );
- }
- if( pAcroForm->GetNeedAppearances() )
- {
- pAcroForm->SetNeedAppearances( false );
- }
- PdfOutputDevice outputDevice( outputfile, true );
- PdfSignOutputDevice signer( &outputDevice );
- PdfString name;
- PdfObject* pExistingSigField = NULL;
- if( field_name )
- {
- name = PdfString( field_name );
- pExistingSigField = find_existing_signature_field( pAcroForm, name );
- if( pExistingSigField && !field_use_existing)
- {
- std::string err = "Signature field named '";
- err += name.GetString();
- err += "' already exists";
- PODOFO_RAISE_ERROR_INFO( ePdfError_WrongDestinationType, err.c_str() );
- }
- }
- else
- {
- char fldName[96]; // use bigger buffer to make sure sprintf does not overflow
- sprintf( fldName, "PodofoSignatureField%" PDF_FORMAT_INT64, static_cast<pdf_int64>( document.GetObjects().GetObjectCount() ) );
- name = PdfString( fldName );
- }
- if( pExistingSigField )
- {
- if( !pExistingSigField->GetDictionary().HasKey( "P" ) )
- {
- std::string err = "Signature field named '";
- err += name.GetString();
- err += "' doesn't have a page reference";
- PODOFO_RAISE_ERROR_INFO( ePdfError_PageNotFound, err.c_str() );
- }
- PdfPage* pPage;
- pPage = document.GetPagesTree()->GetPage( pExistingSigField->GetDictionary().GetKey( "P" )->GetReference() );
- if( !pPage )
- PODOFO_RAISE_ERROR( ePdfError_PageNotFound );
- pTemporaryAnnot = new PdfAnnotation( pExistingSigField, pPage );
- if( !pTemporaryAnnot )
- PODOFO_RAISE_ERROR_INFO( ePdfError_OutOfMemory, "Cannot allocate annotation object for existing signature field" );
- pSignField = new PdfSignatureField( pTemporaryAnnot );
- if( !pSignField )
- PODOFO_RAISE_ERROR_INFO( ePdfError_OutOfMemory, "Cannot allocate existing signature field object" );
- pSignField->EnsureSignatureObject();
- }
- else
- {
- PdfPage* pPage = document.GetPage( annot_page );
- if( !pPage )
- PODOFO_RAISE_ERROR( ePdfError_PageNotFound );
- PdfRect annot_rect;
- if( annot_position )
- {
- annot_rect = PdfRect( annot_left, pPage->GetPageSize().GetHeight() - annot_top - annot_height, annot_width, annot_height );
- }
- PdfAnnotation* pAnnot = pPage->CreateAnnotation( ePdfAnnotation_Widget, annot_rect );
- if( !pAnnot )
- PODOFO_RAISE_ERROR_INFO( ePdfError_OutOfMemory, "Cannot allocate annotation object" );
- if( annot_position && annot_print )
- pAnnot->SetFlags( ePdfAnnotationFlags_Print );
- else if( !annot_position && ( !field_name || !field_use_existing ) )
- pAnnot->SetFlags( ePdfAnnotationFlags_Invisible | ePdfAnnotationFlags_Hidden );
- pSignField = new PdfSignatureField( pAnnot, pAcroForm, &document );
- if( !pSignField )
- PODOFO_RAISE_ERROR_INFO( ePdfError_OutOfMemory, "Cannot allocate signature field object" );
- }
- // use large-enough buffer to hold the signature with the certificate
- signer.SetSignatureSize( min_signature_size );
- pSignField->SetFieldName( name );
- pSignField->SetSignatureReason( PdfString( reinterpret_cast<const pdf_utf8 *>( reason ) ) );
- pSignField->SetSignatureDate( PdfDate() );
- pSignField->SetSignature( *signer.GetSignatureBeacon() );
- // The outputfile != NULL means that the write happens to a new file,
- // which will be truncated first and then the content of the inputfile
- // will be copied into the document, follwed by the changes.
- document.WriteUpdate( &signer, outputfile != NULL );
- if( !signer.HasSignaturePosition() )
- PODOFO_RAISE_ERROR_INFO( ePdfError_SignatureError, "Cannot find signature position in the document data" );
- // Adjust ByteRange for signature
- signer.AdjustByteRange();
- // Read data for signature and count it
- // We seek at the beginning of the file
- signer.Seek( 0 );
- sign_with_signer( signer, cert, pkey );
- signer.Flush();
- }
- catch( PdfError & e )
- {
- if (strcmp(e.what(), "ePdfError_NoPdfFile") == 0)
- {
- throw "Formato de archivo incorrecto.";
- }
- throw (std::string("Error: ") + e.what()).c_str();
- }
- #ifndef PODOFO_HAVE_OPENSSL_1_1
- ERR_free_strings();
- #endif
- if( pSignField )
- delete pSignField;
- if( pTemporaryAnnot )
- delete pTemporaryAnnot;
- return outputfile;
- }
Nota.- Las funciones anteriores fueron modificadas de: PoDoFo
Para poder reutilizar la funcion sign será necesario crear un nuevo archivo de cabecera:
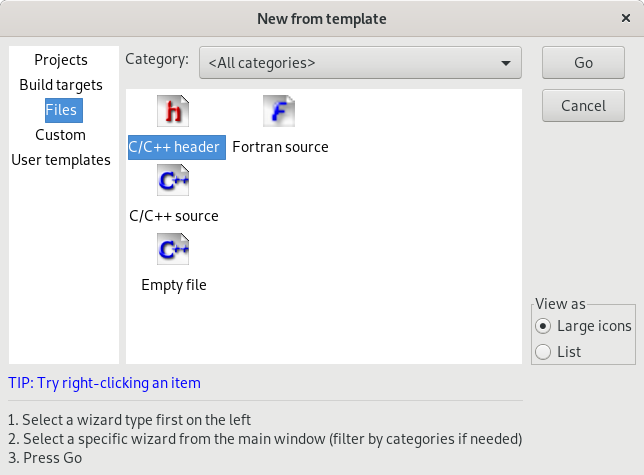
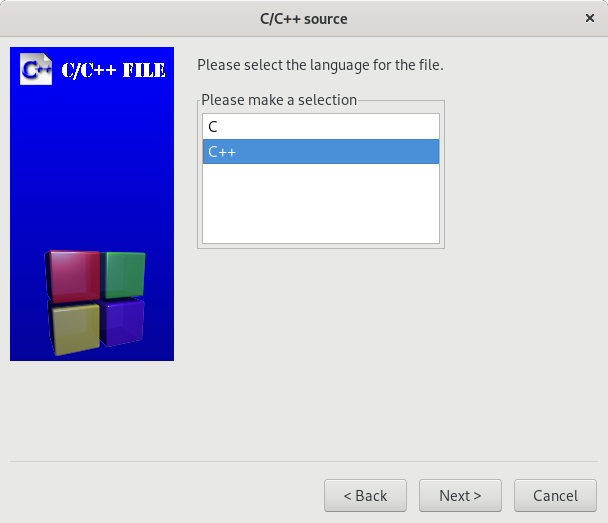
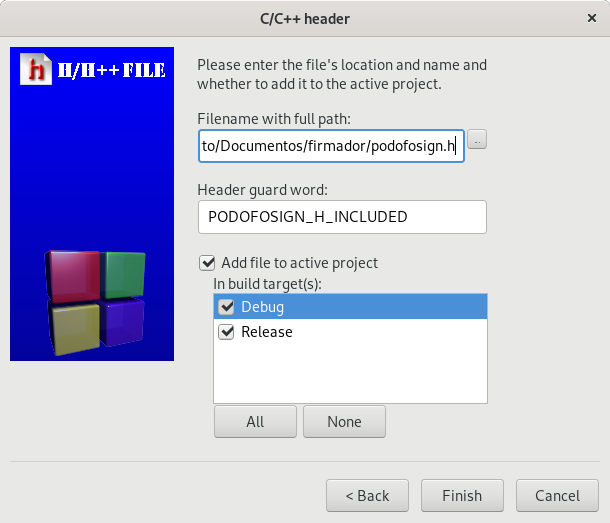
Dentro de la cabecera simplemente habrá que hacer accesible el método sign.
- /*! Método para firmar documentos haciendo uso de PoDoFo. */
- const char* sign(const char *inputfile, const char *filePfx, const char *password);
Y en el método main del archivo main será decirle que archivo y certificado por software utilizar.
- cout << sign("/home/alberto/Documentos/prueba.pdf", "/home/alberto/Documentos/token.p12", "12345678") << endl;
Si se intenta compilar se producirá un error al no estar referenciadas las librerías. Para referenciar elegir la opción Build options del menú contextual.
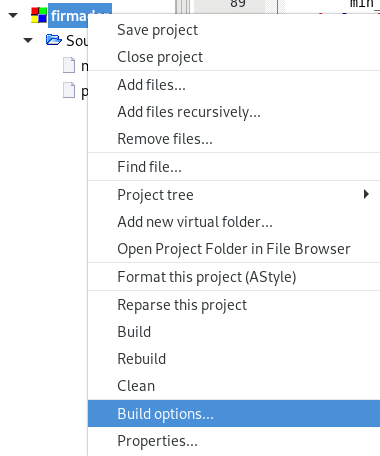
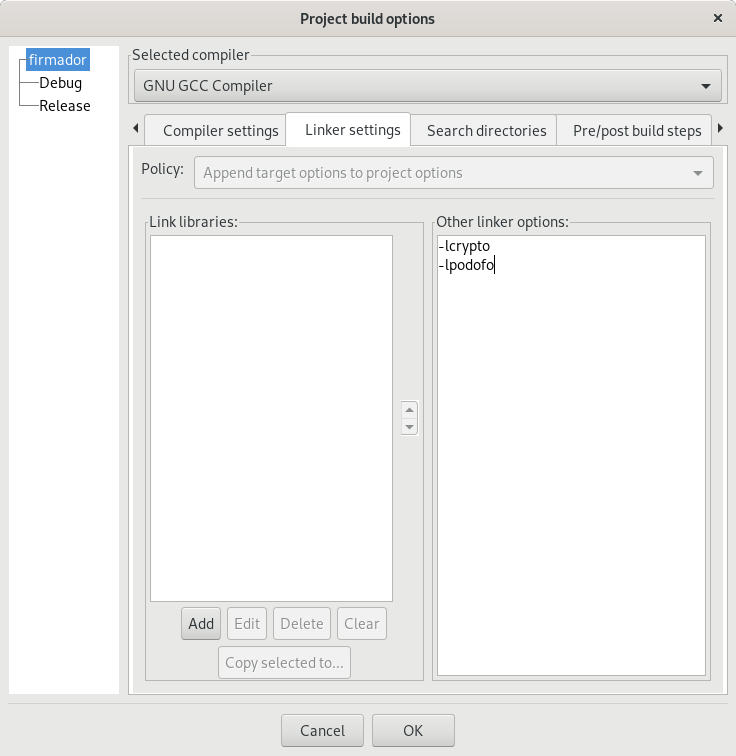
En el panel Other linker options, se especifica las librerías que serán enlazadas, que para este caso son openssl y podofo.
Si se compila y ejecuta se firmará el archivo prueba.pdf y se guardará el archivo firmado en /tmp/firmado.pdf.
Si desea descargar un ejemplo de archivo firmado puede hacerlo a través del siguiente link: Archivo firmado de ejemplo
Si desea descargar el código de ejemplo del programa anterior, puede hacerlo a través del siguiente link: Código de ejemplo